Hello Dev, today we are going to learn that how we can can fetch and set full addresses, live location latitude, longitude or any specific details like city etc.
You can also open this address in Google map with the help of latitude and longitude, So I can say that this article will be help in every situation whenever you deals with map or map activities. download source code: download-source-code-github
also check : custom dialog notification
Step to Address, Location, Latitude and Longitude
- Add Some Line of Code in Manifest File
- Add Google Play Services Dependency
- Design Layout or Views to set details (you can use Toast also for check)
- On Your MainActivity.java write some codes
- Thats it
Details To Fetch Address, Latitude and Longitude
1) Add Manifest permission Code
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
(i) the first two lines of code is responsible for accessing network for the application, if we need something from internet then we need to include INTERNET permissions in out manifest file
(ii) last two lines of permissions are responsible for accessing the maps activity, So if you need any location access of any map type of activity in your application then you must have to add these lines of code
some useful links:
More About: android.permission.INTERNET
More About: Android Permissions
More About: android.permission.ACCESS_FINE_LOCATION
More About: Approximate
Provides an estimate of the device’s location, to within about 1 mile (1.6 km). Your app uses this level of location accuracy when you declare the ACCESS_COARSE_LOCATION permission but not the ACCESS_FINE_LOCATION permission.
Precise
Provides an estimate of the device’s location that is as accurate as possible, usually within about 160 feet (50 meters) and sometimes as accurate as within 10 feet (a few meters) or better. Your app uses this level of location accuracy when you declare the ACCESS_FINE_LOCATION permission.
2) Add Dependency in build.gradle ( module app level )
implementation 'com.google.android.gms:play-services-location:19.0.1'
We need to add android play services location library to enable all classes and methods which is going to use in fetch or accessing the locations.
3) Design Layout.xml (where, details will set or use toast)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp"
android:gravity="center_horizontal"
tools:context=".MainActivity">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/bt_location"
android:text="Get Location"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_marginTop="32dp">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:id="@+id/text_view1"/>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:id="@+id/text_view2"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_marginTop="16dp">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:id="@+id/text_view3"/>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:id="@+id/text_view4"/>
</LinearLayout>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:id="@+id/text_view5"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Open In Map"
android:visibility="invisible"
android:id="@+id/openMap"/>
</LinearLayout>
4) Write the Java Code For Location
(i) Declare views and Locationsprovider class objects
Button btLocation, openMap;
TextView textView1, textView2, textView3, textView4, textView5;
FusedLocationProviderClient fusedLocationProviderClient;
(ii) Assign the views and object define
btLocation = findViewById(R.id.bt_location);
openMap = findViewById(R.id.openMap);
textView1 = findViewById(R.id.text_view1);
textView2 = findViewById(R.id.text_view2);
textView3 = findViewById(R.id.text_view3);
textView4 = findViewById(R.id.text_view4);
textView5 = findViewById(R.id.text_view5);
fusedLocationProviderClient = LocationServices.getFusedLocationProviderClient(this);
(iii) Add click Listener for checking permissions and Load Location
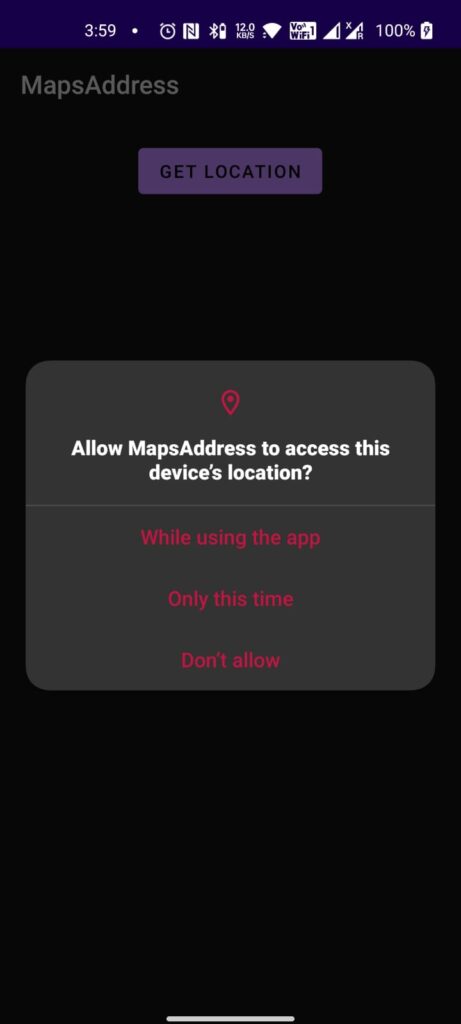
btLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// check permissions
if (ActivityCompat.checkSelfPermission(MainActivity.this,
Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
getLocations();
} else {
ActivityCompat.requestPermissions(MainActivity.this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 44);
}
}
});
(iv) Create getLocations() method
private void getLocations() {
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String[] permissions,
// int[] grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
fusedLocationProviderClient.getLastLocation().addOnCompleteListener(new OnCompleteListener<Location>() {
@Override
public void onComplete(@NonNull Task<Location> task) {
Location location = task.getResult();
if (location != null) {
Geocoder geocoder = new Geocoder(MainActivity.this,
Locale.getDefault());
try {
List<Address> addresses = geocoder.getFromLocation(
location.getLatitude(), location.getLongitude(), 1
);
textView1.setText(Html.fromHtml(
"<font color='#6200EE'><b>Latitude :</b><br></font>"
+ addresses.get(0).getLatitude()
));
textView2.setText(Html.fromHtml(
"<font color='#6200EE'><b>Longitude :</b><br></font>"
+ addresses.get(0).getLongitude()
));
textView3.setText(Html.fromHtml(
"<font color='#6200EE'><b>Country Name :</b><br></font>"
+ addresses.get(0).getCountryName()
));
textView4.setText(Html.fromHtml(
"<font color='#6200EE'><b>Locality :</b><br></font>"
+ addresses.get(0).getLocality()
));
textView5.setText(Html.fromHtml(
"<font color='#6200EE'><b>Address :</b><br></font>"
+ addresses.get(0).getAddressLine(0)
));
} catch (IOException e) {
e.printStackTrace();
}
}
}
});
}
(v) Now Locations Fetched and set Successfully (Full Code)
package com.demo.mapsaddress;
import static androidx.constraintlayout.motion.widget.Debug.getLocation;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.location.Address;
import android.location.Geocoder;
import android.location.Location;
import android.net.Uri;
import android.os.Bundle;
import android.os.Handler;
import android.text.Html;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationServices;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import java.io.IOException;
import java.util.List;
import java.util.Locale;
import java.util.Timer;
import java.util.TimerTask;
public class MainActivity extends AppCompatActivity {
Button btLocation, openMap;
TextView textView1, textView2, textView3, textView4, textView5;
FusedLocationProviderClient fusedLocationProviderClient;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btLocation = findViewById(R.id.bt_location);
openMap = findViewById(R.id.openMap);
textView1 = findViewById(R.id.text_view1);
textView2 = findViewById(R.id.text_view2);
textView3 = findViewById(R.id.text_view3);
textView4 = findViewById(R.id.text_view4);
textView5 = findViewById(R.id.text_view5);
fusedLocationProviderClient = LocationServices.getFusedLocationProviderClient(this);
btLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// check permissions
if (ActivityCompat.checkSelfPermission(MainActivity.this,
Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
getLocations();
} else {
ActivityCompat.requestPermissions(MainActivity.this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 44);
}
}
});
}
private void getLocations() {
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String[] permissions,
// int[] grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
fusedLocationProviderClient.getLastLocation().addOnCompleteListener(new OnCompleteListener<Location>() {
@Override
public void onComplete(@NonNull Task<Location> task) {
Location location = task.getResult();
if (location != null) {
Geocoder geocoder = new Geocoder(MainActivity.this,
Locale.getDefault());
try {
List<Address> addresses = geocoder.getFromLocation(
location.getLatitude(), location.getLongitude(), 1
);
textView1.setText(Html.fromHtml(
"<font color='#6200EE'><b>Latitude :</b><br></font>"
+ addresses.get(0).getLatitude()
));
textView2.setText(Html.fromHtml(
"<font color='#6200EE'><b>Longitude :</b><br></font>"
+ addresses.get(0).getLongitude()
));
textView3.setText(Html.fromHtml(
"<font color='#6200EE'><b>Country Name :</b><br></font>"
+ addresses.get(0).getCountryName()
));
textView4.setText(Html.fromHtml(
"<font color='#6200EE'><b>Locality :</b><br></font>"
+ addresses.get(0).getLocality()
));
textView5.setText(Html.fromHtml(
"<font color='#6200EE'><b>Address :</b><br></font>"
+ addresses.get(0).getAddressLine(0)
));
} catch (IOException e) {
e.printStackTrace();
}
}
}
});
}
}
Open Current Location In Google Map
(i) Set on click listener at any button view see: html string dialog android studio
(ii) Use google map link along with latitude and longitude
openMap.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String strUri = "http://maps.google.com/maps?q=loc:" + addresses.get(0).getLatitude() + "," + addresses.get(0).getLongitude() + " (" + addresses.get(0).getAddressLine(0) + ")";
Intent intent = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse(strUri));
intent.setClassName("com.google.android.apps.maps", "com.google.android.maps.MapsActivity");
Log.d("okkk", strUri);
startActivity(intent);
}
});
Full Code (MainActivity.java)
package com.demo.mapsaddress;
import static androidx.constraintlayout.motion.widget.Debug.getLocation;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.location.Address;
import android.location.Geocoder;
import android.location.Location;
import android.net.Uri;
import android.os.Bundle;
import android.os.Handler;
import android.text.Html;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationServices;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import java.io.IOException;
import java.util.List;
import java.util.Locale;
import java.util.Timer;
import java.util.TimerTask;
public class MainActivity extends AppCompatActivity {
Button btLocation, openMap;
TextView textView1, textView2, textView3, textView4, textView5;
FusedLocationProviderClient fusedLocationProviderClient;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btLocation = findViewById(R.id.bt_location);
openMap = findViewById(R.id.openMap);
textView1 = findViewById(R.id.text_view1);
textView2 = findViewById(R.id.text_view2);
textView3 = findViewById(R.id.text_view3);
textView4 = findViewById(R.id.text_view4);
textView5 = findViewById(R.id.text_view5);
fusedLocationProviderClient = LocationServices.getFusedLocationProviderClient(this);
btLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// check permissions
if (ActivityCompat.checkSelfPermission(MainActivity.this,
Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
getLocations();
} else {
ActivityCompat.requestPermissions(MainActivity.this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 44);
}
}
});
}
private void getLocations() {
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String[] permissions,
// int[] grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
fusedLocationProviderClient.getLastLocation().addOnCompleteListener(new OnCompleteListener<Location>() {
@Override
public void onComplete(@NonNull Task<Location> task) {
Location location = task.getResult();
if (location != null) {
Geocoder geocoder = new Geocoder(MainActivity.this,
Locale.getDefault());
try {
List<Address> addresses = geocoder.getFromLocation(
location.getLatitude(), location.getLongitude(), 1
);
textView1.setText(Html.fromHtml(
"<font color='#6200EE'><b>Latitude :</b><br></font>"
+ addresses.get(0).getLatitude()
));
textView2.setText(Html.fromHtml(
"<font color='#6200EE'><b>Longitude :</b><br></font>"
+ addresses.get(0).getLongitude()
));
textView3.setText(Html.fromHtml(
"<font color='#6200EE'><b>Country Name :</b><br></font>"
+ addresses.get(0).getCountryName()
));
textView4.setText(Html.fromHtml(
"<font color='#6200EE'><b>Locality :</b><br></font>"
+ addresses.get(0).getLocality()
));
textView5.setText(Html.fromHtml(
"<font color='#6200EE'><b>Address :</b><br></font>"
+ addresses.get(0).getAddressLine(0)
));
openMap.setVisibility(View.VISIBLE);
openMap.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String strUri = "http://maps.google.com/maps?q=loc:" + addresses.get(0).getLatitude() + "," + addresses.get(0).getLongitude() + " (" + addresses.get(0).getAddressLine(0) + ")";
Intent intent = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse(strUri));
intent.setClassName("com.google.android.apps.maps", "com.google.android.maps.MapsActivity");
Log.d("okkk", strUri);
startActivity(intent);
}
});
} catch (IOException e) {
e.printStackTrace();
}
}
}
});
}
}
Fetch or Load Live Location Android Studio
To fetch or load live location in android we need to understand the concept behind the live location, A simple term is used here and that is fetch location at every 3 second.
If you can do this we can easily find the live location, but problem is how can we fetch or load location on every particular second, So we solve this problem by setting looper and handler with help of onPause and onResume by overriding the methods according to out needs
(i) We are using same method for fetching the locations (getLocations)
(ii) used same UI and codes
(iii) Lets take 10sec (fetch location on every 10 seconds)
@Override
protected void onResume() {
handler.postDelayed(runnable = new Runnable() {
public void run() {
handler.postDelayed(runnable, delay);
getLocations();
Toast.makeText(MainActivity.this, "call Address every 10 sec",
Toast.LENGTH_SHORT).show();
}
}, delay);
super.onResume();
}
@Override
protected void onPause() {
super.onPause();
handler.removeCallbacks(runnable); //stop handler when activity not visible super.onPause();
}
Full Code (MainActivity.java)
package com.demo.mapsaddress;
import static androidx.constraintlayout.motion.widget.Debug.getLocation;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.location.Address;
import android.location.Geocoder;
import android.location.Location;
import android.net.Uri;
import android.os.Bundle;
import android.os.Handler;
import android.text.Html;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationServices;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import java.io.IOException;
import java.util.List;
import java.util.Locale;
import java.util.Timer;
import java.util.TimerTask;
public class MainActivity extends AppCompatActivity {
Button btLocation, openMap;
TextView textView1, textView2, textView3, textView4, textView5;
FusedLocationProviderClient fusedLocationProviderClient;
Handler handler = new Handler();
Runnable runnable;
int delay = 10000;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btLocation = findViewById(R.id.bt_location);
btLocation (View.INVISIBLE);
openMap = findViewById(R.id.openMap);
textView1 = findViewById(R.id.text_view1);
textView2 = findViewById(R.id.text_view2);
textView3 = findViewById(R.id.text_view3);
textView4 = findViewById(R.id.text_view4);
textView5 = findViewById(R.id.text_view5);
fusedLocationProviderClient = LocationServices.getFusedLocationProviderClient(this);
}
private void getLocations() {
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String[] permissions,
// int[] grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
fusedLocationProviderClient.getLastLocation().addOnCompleteListener(new OnCompleteListener<Location>() {
@Override
public void onComplete(@NonNull Task<Location> task) {
Location location = task.getResult();
if (location != null) {
Geocoder geocoder = new Geocoder(MainActivity.this,
Locale.getDefault());
try {
List<Address> addresses = geocoder.getFromLocation(
location.getLatitude(), location.getLongitude(), 1
);
textView1.setText(Html.fromHtml(
"<font color='#6200EE'><b>Latitude :</b><br></font>"
+ addresses.get(0).getLatitude()
));
textView2.setText(Html.fromHtml(
"<font color='#6200EE'><b>Longitude :</b><br></font>"
+ addresses.get(0).getLongitude()
));
textView3.setText(Html.fromHtml(
"<font color='#6200EE'><b>Country Name :</b><br></font>"
+ addresses.get(0).getCountryName()
));
textView4.setText(Html.fromHtml(
"<font color='#6200EE'><b>Locality :</b><br></font>"
+ addresses.get(0).getLocality()
));
textView5.setText(Html.fromHtml(
"<font color='#6200EE'><b>Address :</b><br></font>"
+ addresses.get(0).getAddressLine(0)
));
openMap.setVisibility(View.VISIBLE);
openMap.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String strUri = "http://maps.google.com/maps?q=loc:" + addresses.get(0).getLatitude() + "," + addresses.get(0).getLongitude() + " (" + addresses.get(0).getAddressLine(0) + ")";
Intent intent = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse(strUri));
intent.setClassName("com.google.android.apps.maps", "com.google.android.maps.MapsActivity");
Log.d("okkk", strUri);
startActivity(intent);
}
});
} catch (IOException e) {
e.printStackTrace();
}
}
}
});
}
@Override
protected void onResume() {
handler.postDelayed(runnable = new Runnable() {
public void run() {
handler.postDelayed(runnable, delay);
getLocations();
Toast.makeText(MainActivity.this, "call Address every 10 sec",
Toast.LENGTH_SHORT).show();
}
}, delay);
super.onResume();
}
@Override
protected void onPause() {
super.onPause();
handler.removeCallbacks(runnable); //stop handler when activity not visible super.onPause();
}
}